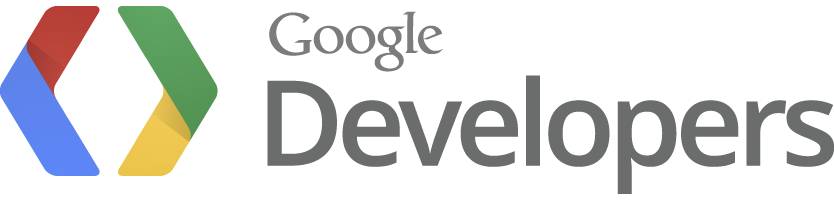
·
navigator.onLine window.(ononline|onoffline)
.circle { width: 300px; height: 300px; } div { display: ; width: (100% - 4em); height: (100% - 4em); border-radius: 50%; : center; : center; } div:hover { border-radius: 0; }
Aplique efeitos e filtros a qualquer elemento DOM:
video, img { : grayscale(0.5) blur(10px); }
Leia mais em blog post.
.cssshade:hover { -webkit-filter: custom(url(shaders/vertex/crumple.vs) mix(url(shaders/fragment/crumple.fs) multiply source-atop), 50 50 border-box, transform perspective(1000) scale(1) rotateX(0deg) rotateY(0deg) rotateZ(0deg), amount 1, strength 0.4, lightIntensity 1); }
Confirme que o flag CSS Shaders
está ativado em chrome://flags e passe o mouse sobre o código acima.
function startWorker(settings) { var myWorker = new Worker('scripts/worker.js'); myWorker.addEventListener("message", workerListener, false); myWorker.postMessage(settings); }
self.addEventListener('message', function(e) { doSomeWork(); } function doSomeWork() { importScripts('js/script1.js'); ... postMessage(result); }
Abrindo um filesystem:
window.( TEMPORARY, // PERSISTENT vs. TEMPORARY 1024 * 1024, // tamanho (bytes) requerido initFs, // callback de sucesso opt_errorHandler // callback de erro );
var xhr = new XMLHttpRequest(); xhr.open('GET', '/path/to/image.png', true); xhr.responseType = 'arraybuffer'; xhr.onload = function(e) { }; xhr.send();
var xhr = new XMLHttpRequest(); xhr.open('GET', '/path/to/image.png', true); xhr.responseType = 'arraybuffer'; xhr.onload = function(e) { window.(TEMPORARY, 1024 * 1024, function(fs) { }, onError); }; xhr.send();
var xhr = new XMLHttpRequest(); xhr.open('GET', '/path/to/image.png', true); xhr.responseType = 'arraybuffer'; xhr.onload = function(e) { window.(TEMPORARY, 1024 * 1024, function(fs) { fs.root.getFile('image.png', {create: true}, function(fileEntry) { }, onError); }, onError); }; xhr.send();
var xhr = new XMLHttpRequest(); xhr.open('GET', '/path/to/image.png', true); xhr.responseType = 'arraybuffer'; xhr.onload = function(e) { window.(TEMPORARY, 1024 * 1024, function(fs) { fs.root.getFile('image.png', {create: true}, function(fileEntry) { fileEntry.createWriter(function(writer) { writer.onwriteend = function(e) { ... }; writer.onerror = function(e) { ... }; writer.write(new Blob([xhr.response], {type: 'image/png'})); }, onError); }, onError); }, onError); }; xhr.send();
filesystem:
) URL:filesystem:http://example.com/temporary/image.png
var img = document.createElement('img'); img.src = fileEntry.toURL(); document.body.appendChild(img);
FileEntry
de uma URL filesystem:
?window.(img.src, function(fileEntry) { ... });
var xhr = new XMLHttpRequest(); xhr.open('GET', '/path/to/image.png', true); xhr.responseType = 'arraybuffer'; xhr.onload = function(e) { window.(TEMPORARY, 1024 * 1024, function(fs) { fs.root.getFile('image.png', {create: true}, function(fileEntry) { fileEntry.createWriter(function(writer) { writer.onwriteend = function(e) { ... }; writer.onerror = function(e) { ... }; writer.write(new Blob([xhr.response], {type: 'image/png'})); }, onError); }, onError); }, onError); }; xhr.send();
Biblioteca que implementa comandos UNIX (ls, cp, mv)
var filer = new Filer(); filer.init({persistent: false, size: 1024 * 1024}, function(fs) {...}, onError); filer.ls('path/to/some/dir/', function(entries) { ... }, onError); filer.cp('file.txt', '/path/to/folder', 'newname.txt', function(entry) { // entry.fullPath == '/path/to/folder/newname.txt' }, onError); var b = new Blob(['body { color: red; }'], {type: 'text/css'}); filer.write('styles.css', {data: b, type: b.type}, function(entry, writer) { ... }, onError);
Forçar downloads do servidor:
Content-Disposition: attachment; filename="MyLogo.png";
Atributo download
para fazer download de arquivos ao invés de navegar:
<a href="filesystem:http://example.com/temporary/image.png" download="image.png">download me</a>
function downloadLink(name, content, mimetype) { var a = document.createElement('a'); a.href = window.( new Blob([content], {type: mimetype})); a.download = name; a.textContent = 'Download pronto'; document.body.appendChild(a); } downloadLink('MyNovel.txt', document.querySelector('textarea').textContent, 'text/plain');
Device APIs WG: www.w3.org/2009/dap/
navigator.onLine
/ navigator.connection
( conectividade de rede )<input type="text" x-webkit-speech lang="pt_BR">
WebRTC: acesso à câmera e ao microfone sem plugins
navigator.({audio: true, video: true}, function(stream) { var video = document.querySelector('video'); video.src = window.(stream); }, function(e) { console.log(e); });
<video autoplay controls></video>
<input type="button" value="♦" onclick="record(this)"> <input type="button" value="◼" onclick="stop(this)">
var localMediaStream, recorder; var record = function(button) { recorder = localMediaStream.record(); }; var stop = function(button) { localMediaStream.stop(); recorder.getRecordedData(function(blob) { // Upload blob using XHR2. }); };
getUserMedia()
File
, Blob
, ou ArrayBuffer
!var socket = new WebSocket('ws://example.com/sock', ['dumby-protocol']); socket.binaryType = 'blob'; // or 'arraybuffer' socket.onopen = function(e) { window.setInterval(function() { if (socket.bufferedAmount == 0) { socket.send(new Blob([blob1, blob2])); } }, 50); // executa a cada 50ms apenas }; socket.onmessage = function(e) { document.querySelector('img').src = (e.data); };
Meta tag:
<meta http-equiv="X-UA-Compatible" content="IE=Edge,chrome=1">
Header:
X-UA-Compatible: IE=Edge,chrome=1